We will learn how to automate Docker builds using Jenkins and Deploy into Kubernetes Cluster in AWS Cloud. We will use kubectl command to deploy Docker images into EKS cluster. We will use Python based application. I have already created a repo with source code + Dockerfile. The repo also have Jenkinsfile for automating the following:
- Automating builds using Jenkins
- Automating Docker image creation
- Automating Docker image upload into Elastic container registry
- Automating Deployments to Kubernetes Cluster using kubectl CLI plug-in
- Automating Docker image creation
- Automating Docker image upload into Elastic container registry
- Automating Deployments to Kubernetes Cluster using kubectl CLI plug-in
Pre-requisites:
1. EKS Cluster is setup and running. Click here to learn how to create EKS cluster.
1. EKS Cluster is setup and running. Click here to learn how to create EKS cluster.
2. Jenkins Master is up and running.
3. Install Docker in Jenkins.
4. Docker, Docker pipeline and Kubectl CLI plug-ins are installed in Jenkins
The Code for this video is here:
Make sure you fork my repo https://github.com/akannan1087/myPythonDockerRepo
and make necessary changes in eks-deploy-from-ecr.yaml file after you fork into your account.
Step #1 - Create Credentials for connecting to EKS cluster using Kubeconfig
Go to Jenkins UI, click on Credentials -->
Click on Global credentials
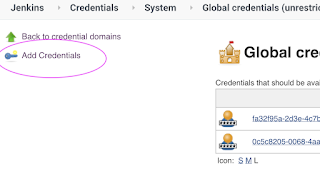
use secret file from drop down.
Click on Global credentials
Click on Add Credentials
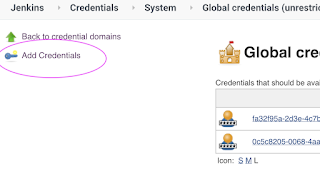
use secret file from drop down.
execute the below command to login as jenkins user.
sudo su - jenkins
you should see the nodes running in EKS cluster.
kubectl get nodes
cat /var/lib/jenkins/.kube/config
Open your text editor or notepad, copy and paste the entire content and save in a file.
We will upload this file.
Enter ID as K8S and choose File and upload the file and save.
Step # 2 - Create a pipeline in Jenkins
Step # 3 - Copy the pipeline code from below
Make sure you change values as per your settings highlighted in yellow below:
Make sure you change values as per your settings highlighted in yellow below:
pipeline {
agent any
environment {
registry = "account_id.dkr.ecr.us-east-1.amazonaws.com/coachak/my-docker-repo"
}
stages {
stage('checkout') {
steps {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], extensions: [], userRemoteConfigs: [[url: 'https://github.com/akannan1087/myPythonDockerRepo']]])
}
}
stage ("build image")
{
steps {
script {
dockerImage = docker.build registry
dockerImage.tag("$BUILD_NUMBER")
}
}
}
stage ("upload ECR") {
steps {
script {
sh "aws ecr get-login-password --region us-east-2 | docker login --username AWS --password-stdin account_id.dkr.ecr.us-east-2.amazonaws.com"
sh 'docker push account_id.dkr.ecr.us-east-1.amazonaws.com/coachak/my-docker-repo:$BUILD_NUMBER'
}
}
}
// Avoid latest tag image and pass build ID dynamically from Jenkins pipeline
stage('K8S Deploy') {
steps{
script {
withKubeConfig([credentialsId: 'K8S', serverUrl: '']) {
echo "Current build number is: ${env.BUILD_ID}"
// Replace the placeholders in the deployment.yaml file
sh """
sed -i 's/\${BUILD_NUMBER}/${env.BUILD_ID}/g' k8s-deployment.yaml
"""
sh ('kubectl apply -f k8s-deployment.yaml -n springboot-app-ns')
}
}
}
}
}
}
Step # 4 - Build the pipeline
Step # 5 - Verify deployments to EKS
kubectl get pods
kubectl get deployments
kubectl get services
Steps # 6 - Access Python App in K8S cluster
Once deployment is successful, go to browser and enter above load balancer URL
You should see page like below:
No comments:
Post a Comment